The Scandit SDK supports add-on codes (also known as Extension Codes) for EAN-8, EAN-13, UPC-A and UPC-E codes. These codes encode additional product data like an issue number, date or price. There is a two (EAN-2/two-digit add-on) and a five digit (EAN-5/five-digit add-on) version.
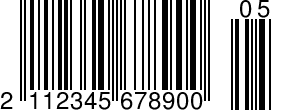
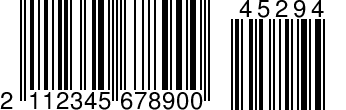
Enable add-on codes in the SDK
- Enable both the main symbologies (e.g. EAN-13) and the add-on symbology (e.g. two-digit add-on) that you want to scan.
- Set the number of codes to scan in a frame to 2.
Accumulate scan results until two codes were scanned that fulfill the following conditions:
- One corresponds to the main symbology
- The other one corresponds to a symbology extension
The data of the two scanned codes is then returned as two separate results. Be aware that it can happen that only the main code is found in a particular frame. Isolated extension codes are never returned as a result.
Linux
Settings setup for EAN-13/UPC-A codes with a 3 or 5 digit add-on on Linux:
The Add-On code and the main code (EAN13/UPCA, EAN8, or UPCE) are returned as two separate codes and the result needs to be combined from the scan session. A possible implementation of the result collection can look like this: